Grab your Unity assets at discounts!
The Singleton Pattern in Unity – A Detailed Guide
The Singleton pattern is an essential tool for structuring Unity projects efficiently. This tutorial provides a step-by-step guide to implementing Singletons in Unity, covering both basic and advanced techniques. You’ll learn how to apply Singleton in GameManager, AudioManager, UIManager, and more while avoiding common pitfalls like tight coupling. We also explore thread-safe Singletons, generic implementations, and event-driven approaches to make your projects more scalable and maintainable. 🚀 Perfect for Unity developers looking to improve project architecture and performance!
Indie Impulse
2/7/20252 min read
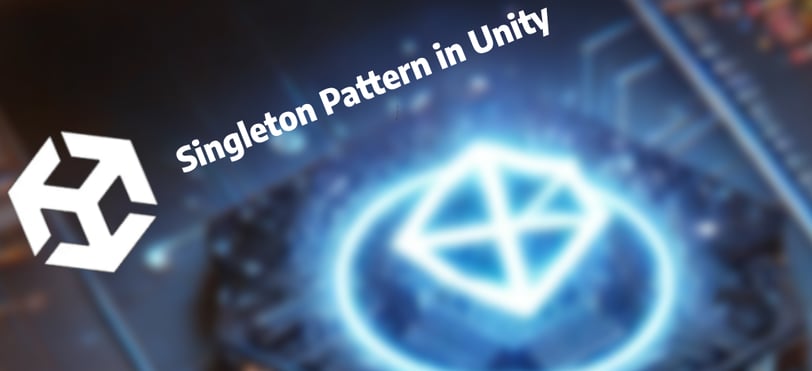
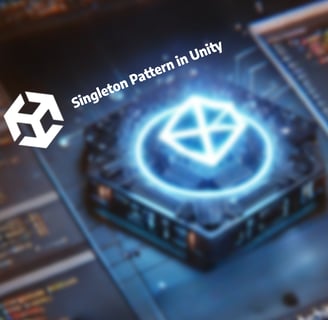
Introduction
The Singleton pattern is one of the most widely used design patterns in Unity game development. It ensures that only one instance of a class exists, providing a centralized and global access point. This is particularly useful for game-wide managers like GameManager, AudioManager, and UIManager.
In this tutorial, we will cover:
✅ What the Singleton Pattern is
✅ When to use it in Unity
✅ A step-by-step guide to implementing a Singleton in Unity
✅ Advanced Singleton techniques
✅ Common pitfalls and best practices
✅ Real-world examples
What is the Singleton Pattern?
The Singleton pattern restricts a class to having only one instance while providing global access to it. It ensures that all parts of the game interact with the same instance rather than creating multiple copies, avoiding inconsistencies.
✔️ Ensures a single instance exists
✔️ Provides global access to the instance
✔️ Prevents duplicate objects from being created
✔️ Useful for game-wide systems like settings, sound management, and data persistence
When to Use Singleton in Unity?
Singletons are best used for:
✔️ GameManager: Managing game states, score tracking, and global settings.
✔️ AudioManager: Handling background music, sound effects, and volume levels.
✔️ UIManager: Managing different UI panels and animations.
✔️ SaveManager: Persisting player progress and preferences.
✔️ InputManager: Centralizing input handling for different controllers.
✔️ NetworkManager: Handling network connections in multiplayer games.
However, overusing singletons can lead to tightly coupled code, so use them wisely.
Implementing a Singleton in Unity
Step 1: Basic Singleton Implementation
Here's a simple Singleton implementation for a GameManager class:
Breaking Down the Code:
public static GameManager Instance { get; private set; }
A static property that stores the single instance.
private void Awake()
Ensures only one instance exists.
if (Instance == null) { Instance = this; DontDestroyOnLoad(gameObject); }
If no instance exists, assign this as the instance and make it persist across scenes.
else { Destroy(gameObject); }
If an instance already exists, destroy the duplicate.
Singleton with Lazy Initialization (Non-MonoBehaviour)
For non-MonoBehaviour classes, use a static property:
This ensures the instance is created only when first accessed.
Advanced Singleton Techniques
Thread-Safe Singleton (For Multithreading)
For cases where multithreading is involved, ensure thread safety using lock:
Generic Singleton for Reusability
To avoid duplicating singleton logic, create a generic singleton base class:
Usage:
This allows you to create different managers without rewriting singleton logic.
Singleton with Event System
To enhance functionality, combine Singleton with an event-driven system:
Usage:
Common Pitfalls & Best Practices
Avoid Overusing Singletons
Over-reliance on singletons can lead to tightly coupled code.
Makes unit testing more difficult.
Use Dependency Injection Where Possible
Inject dependencies instead of accessing them via a singleton.
Ensure Proper Cleanup
DontDestroyOnLoad can cause issues if objects persist unintentionally.
Destroy instances explicitly when necessary.
Be Mindful of Multi-Scene Setups
Ensure singletons don’t cause issues when transitioning between scenes.
Practical Implementations of the Singleton Pattern
Audio Manager Singleton
GameManager Singleton with Score Tracking
Conclusion
The Singleton pattern is a powerful tool for managing global game systems efficiently. However, it should be used cautiously to avoid potential drawbacks like tight coupling and difficult testing. By following best practices and advanced techniques, you can make the most out of Singletons in Unity.